Online Resources
Extra Reading
Basics
Where do you include JavaScript?
- In head
- In body
- End of body so it doesn't interfere with loading HTML
- External file (.js)<script src="title.js"></script>
- Make sure .js file is in the same directory as .html you are linking to
Comments & Displaying JavaScript
- alert(""); Pop-up box on page
- console.log(""); Records in console/inspector
- document.write(""); Writes on the page
- document.getElementById("idName");
- Single line comment: //
- Multi line comment: /* line 1
- line 2 */
- To comment out something already typed: Highlight → cmd /
Google Dev Tool Tips
Console allows you to run one line of code at a time to test it out.
Snippets inside Dev Tools allows you to write an entire section of code before running it.
- Sources → Snippets → New Snippet
To clear the console quickly → cmd + K
5 Primitive Data Types
- Numbers: 4, 9.3, -10
- Strings: text inside quotes "Hello, World!", "42"
- Boolean: only 2 types: true / false
- Null
- Undefined
Undefined
- Variables that are declared, but not initialized are undefined
- Example: var name;
- Example: var age;
Null
- Null is "explicitly nothing"
- var currentPlayer = "charlie";
- But if, currentPlayer = null; → Game Over
Variables
Variables are simply containers with names. You can put data into these containers and then refer to the data by calling the container name.
Convention for Naming Variables
- Name it something logical
- Start with a letter, _(underscore) or $
- Can contain numbers after first digit
- Cannot contain spaces
- Can only use numbers, letters, underscore and $
- Case sensitive
- Convention is to name variables using camelCase.
- camelCase: 1st letter lowercase, following words are capitalized: yourVariableName
- Other types:
- snake_case: underscores between words
- kebab-case: dash between words
var - let - const
- var declarations are globally scoped or function scoped while let and const are block scoped.
- var variables can be updated and redeclared within its scope.
- let variables can be updated, but not redeclared.
- const variables can neither be updated nor redeclared.
Declaring a Variable: let
Basic Syntax:
- Make a variable called "age" and give it the value of 55;
- let age = 55;
- Recall Variables
- let hens = 4;
- let roosters =li2;
- hens + roosters
- → 6
- Update Values
- let hens = 4;
- Update - Wrong syntax:
- hens - 1;
- hens;
- → 4...this does not change the value stored in hens
- Update - Correct syntax:
- hens = hens - 1;
- hens;
- → 3
- Shortcut to updating values
Instead of score = score + 10; you can use score += 10;
Declaring a Variable: var
- (declare only) var nameOfVariable;
OR (declare while setting an initial value) var nameOfVariable = value; - Two ways to declare a variable
- Individually
- var money;
- var name;
- Multiple with same var keyword separated with comma
- var money, name;
- Once it's declared you can change the data by just inputing name = ; (no var)
- var number1 = 10;
- var number2 = 20;
- number1 = 5;
- var total = number1 + number2;
- alert(total);
- Answer: 25
- You can declare a variable without setting an initial value
- var number1;
- number1 = 10;
const Variable
const works just like let, except you CANNOT change the value
JavaScript Methods
- alert(" ");
- Creates a popup window for user
- console.log(" ");
- Prints to JavaScript console
- If log is not open then the user will not see it--really just a tool for developers
- prompt(" ");
- Creates a popup window that has text and an input field for the user
- This is useful for developer because you can store their answer in a variable
Numbers & Arithmetic in JavaScript
- + Addition
- - Subtraction
- * Multiplication
- / Division
- % Modulo (Mod)
- Remainder operator
- 10 % 3 = 1
- 3 goes into 10, 3 times remainder 1, so the answer is 1
- This is useful when determining whether a number is odd or even (_%2)
- You can do the calculation inside of the variable
- var x = 10 + 5;
- alert(x);
- You can set separate variables and then do calculations inside the alert
- var x = 9;
- var y = 5;
- alert (x*y);
- When doing calculations the order of operations is in effect:
- P E M (or) D A (or) S
- P = parentheses
- E = exponents
- M = multiply or D = divide (left to right)
- A = add or S = subtract (left to right)
- Use + - to increment and decrement
- var a = 10;
- ++a; → 11
Boolean Logic
Everything starts with the idea that a statement is either True or False.
Then we can combine those initial statements to create more complex statements that also evaluate to True or False.
Comparison Operators
Assuming x = 5
Operator
>
>=
<
<=
==
!=
===
!==
Name
greater than
greater than/equal
less than
less than/equal
equal value, not comparing type
not equal to
equal value & type
not equal value nor equal type
Example
x > 10
x >= 5
x < -50
x <= 100
x == "5"
x != "b"
x === "5"
x !== "5"
Result
false
true
false
true
true
true
false
true
Logical Operators: AND, OR, NOT
Assume x = 5, y = 9
Operator
&&
||
!
Name
AND
OR
NOT
Example
x < 10 && x !== 5
y > 9 || x === 5
! ( x === y )
Result
false
true
true
Operator Precedence
> and < run before && and ||
! has higher precedence than && and ||
&& has higher precedence than ||
Equality Operators
== vs. ===
(It is better to use === than ==)
var x = 99;
x == "99" → true
x === "99" → false
var y = null;
y == undefined → true
y === undefined → false
Truthy & Falsey Values
Values that aren't actually true or false are still inherently "truthy" or "falsey" when evaluated in a boolean context.
Falsey Values
- false
- 0
- ""
- null
- undefined
- NaN
Everything else is truthy
JavaScript Strings
MDN Docs: String - Scroll down to Escape Notation for more info
A string is a selection of text: a character, a word, or a paragraph
- Can use single or double quotes
- If you want quotes inside of a string then start with double and use single inside, or vice versa
- When setting a variable as a paragraph it can be hard to read it in the console
- \n will start a new line
- \" text here will have quotes \"
- 'She said \"I'm not going to quit\"'
- \\ will show one \ within text
- Concatenation: put two strings together
- "charlie" + "brown" → "charliebrown"
- To escape characters: start with \" This allows you to type characters that would normally cause a problem in the code
- "This is a backslash: \\" → "This is a backslash: \"
- "She said \"goodbye!\"" → "She said "goodbye!"
- Access individual characters using [] and an index (number in middle)--*Remember JavaScript starts counting at 0!*
- "hello"[0] → "h"
- "hello"[4] → "o"
- So .length starts counting from 1, and index starts counting from 0.
- To get the last character of a string you .length first, but subtract 1 because index starts counting at 0
- let str = "hello";
- str[str.length-1] → o
String Methods
.toUpperCase() - Convert string to uppercase
- <script>
- var string = "Learning Javascript";
- var newString = string.toUpperCase();
- alert(newString);
- </script>
- → "LEARNING JAVASCRIPT"
.toLowerCase() - Convert string to lowercase
- string.toLowerCase()
- → "learning javascript"
.replace() - Replace words in string
- var string = "I want to learn Javascript to build websites";
- var newString = string.replace("websites", "games");
- First one in list is the one you want to replace, and the second one is what you want to replace it with
- alert(newString);
- → "I want to learn Javascript to build games"
.split("") - Split strings into individual characters
- var string = "Learning Javascript";
- var newString = string.split("");
- alert(newString);
- → L,e,a,r,n,i,n,g, ,J,a,v,a,s,c,r,i,p,t
.split(" ") - Split strings into individual words
- var string = "Learning Javascript";
- var newString = string.split(" ");
- Space in the middle of the quotes breaks the string where the spaces are
- alert(newString);
- → "Learning,Javascript"
.split(" ")[x] - Split string into individual words, Access word by using index number
- var string = "Learning Javascript";
- var newString = string.split(" ");
- alert(newString[0]);
- → "Learning"
.concat() - Join strings together
- var string = "Learning Javascript";
- var newString = string.split(" ");
- var joinedString = newString[0].concat(newString[1]);
- alert(joinedString);
- → "LearningJavascript"
.trim() - Trim extra spaces
- let greeting = " hello there ";
- greeting.trim()
- → "hello there"
.slice(x,y) - Slice method
- var string = "Learning Javascript";
- var newString = string.slice(2,6);
- Numbers inside parentheses designate starting position of slice and everything up to (but not including) the last number listed. Don't forget index counting starts at 0.
- alert(newString);
- → "arni" (Shows index 2,3,4,5 → stops at index 6)
- A tip to figuring out how many characters you will get in solution is to subtract the first number listed from the second number. 6-2=4, 4 characters
- You can choose to only list one number inside of the (). If you do, it will start at that index position and give you everything from there to the end of the string.
- let sport = "baseball";
- sport.slice(4); → "ball"
.indexOf() - Look for Substring inside of String
- indexOf() method looks for a substring inside of a string. If found it will return the index position of where the substring begins.
- It will return -1 if the string is not found.
- If you look for something that occurs more than once in the string, it will only return the first instance.
- let tvShow = 'catdog';
- tvShow.indexOf('cat'); → 0
- tvShow.indexOf('dog'); → 3
- tvShow.indexOf('z'); → -1 (not found)
.substring(x,y) - Substring method
- The substring() method extracts the characters from a string, between two specificed indices, and returns the new sub string.
- This method extracts the characters in a string between "start" and "end", but not including "end" itself.
- If "start" is greater than "end", this method will swap the two arguments, meaning str.substring(1,4) == (str.substring(4,1).
- If either "start" or "end" is less than 0, it is treated as if it were 0.
Begin the extraction at position 2, and extract the rest of the string:
- var string = "Learning Javascript";
- var newString = string.substring(2);
- → arning Javascript
If "start" is greater than "end", it will swap the two arguments:
- var string = "Learning Javascript";
- var newString = string.substring(4,1); (This is the same as if it said (1,4)
- → ear
Extract only the first character
- var string = "Learning Javascript";
- var newString = string.substring(0,1);
- → L
Extract only the last character
- var string = "Learning Javascript";
- var newString = string.substring(str.length - 1, str.length);
- → t
String Template Literals
MDN Docs: Template Literals (Template strings)
JavaScriptTutorial.net: JavaScript Template Literals in Depth
Template literals are string literals allowing embedded expressions. You can use multi-line strings and string interpolation features with them.
Whatever is inside `${x+y}` will be run first.
Syntax:
- Important! Use back-ticks, not single-quotes for these. (Back-tick key is usually above the tab key)
- Also, use dollar sign and curly braces {}
Old way using concatenation:
- let animal = "pig";
- let sound = "oink";
- animal + " says " + sound + "!"
- → "pig says oink!"
New way using Template Literals
- let animal = "pig";
- let sound = "oink";
- `${animal} says ${sound}!
- → "pig says oink!"
Also, allows for multiline string: a string that can span multiple lines (without \n or < br>)
- let p =
`This text
can
span multiple lines`;
String formatting: the ability to substitute part of the string for the values of variables or expressions. This feature is also called string interpolation.
- `GAME OVER ${username.toUpperCase()}`
- → "GAME OVER ZIGGY31"
Another example:
- let item = 'cucumbers';
- let price = 1.99;
- let quantity = 4;
- `You bought ${quantity} ${item}, total price: $${price*quantity}`;
- → "You bought 4 cucumbers, total price: $7.96"
Functions
A block of code you call when required. This way you don't have to write the code more than once. You just write it once inside a function, and call the function when you need it.
Stanford Karel Function Practice
Declare a function:
- function doSomething() {
console.log("Hello, World");
}
Call a function:
- doSomething();
- Don't need the "function" at the beginning
- Ends with semicolon
Arguments & Parameters in Functions
The parameters of a function/method describe to you the value that it uses to calculate its result. The arguments of a function are the values assigned to these parameters during a particular call of the function/method.
Medium: Anatomy of a Javascript Function Part 1
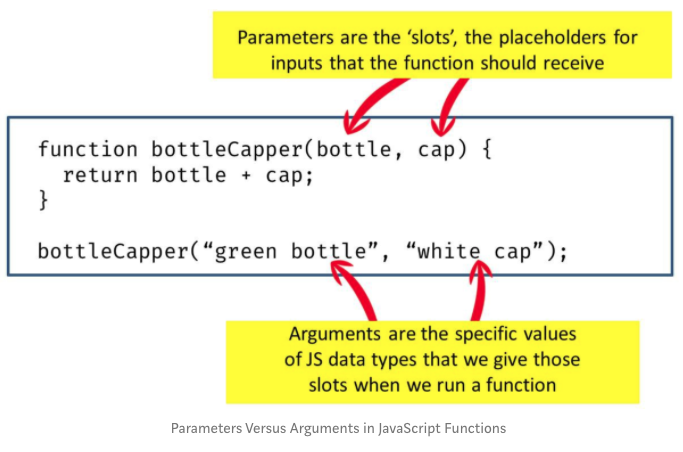
Example:
- Parameters: (length, width)
- Arguments: (9,2)
- function area(length, width) {
- console.log(length * width);
- }
- area(9,2);
- → 18
Number of parameters needs to match number of arguments
- Parameters: (person1, person1, person3)
- Arguments: ("Harry", "Ron", "Hermione")
- function greet(person1, person2, person3) {
- console.log("Hi " + person1);
- console.log("Hi " + person2);
- console.log("Hi " + person3);
- }
- greet("Harry", "Ron", "Hermione");
- → "Hi Harry"
- → "Hi Ron"
- → "Hi Hermione"
The Return Keyword
We can use the return keyword to output a value from a function.
- Return → allows your output to be used in further code--can be saved in a variable, etc.
- Console.log → just prints for the developer--can't use the output for anything else.
This example shows how the return results are saved in a variable
→This function capitalizes the 1st char in a string
- function capitalize(str) {
- return str.charAt(0).toUpperCase() + str.slice(1);
- }
- var city = "paris";
- var capital = capitalize(city);
- → "Paris"
The return keyword stops execution of a function
The return keyword stops execution and returns to the calling function. When a return statement is executed, the function is terminated immediately at that point, regardless of whether it's in the middle of a loop, etc.
- function capitalize(str) {
- if(typeof str === "number") {
- return "that's not a string";
- }
- return str.charAt(0).toUpperCase() + str.slice(1);
- }
- var num = 37;
- var capital = capitalize(num);
- → "that's not a string"
- var city = "paris";
- var capital = capitalize(city);
The above function stopped after detecting a number, so the rest of the function was not executed.
Function Expression vs. Function Declaration
A function expression is very similar to and has almost the same syntax as s function declaration. The main difference between a function expression and a function declaration is the function name, which can be omitted in function expressions to create anonymous functions.
- Function Expression
- var capitalize = function(str) {
- return str.charAt(0).toUpperCase() + str.slice(1);
- }
- Function Declaration
- function capitalize(str) {
- return str.charAt(0).toUpperCase() + str.slice(1);
- }
Math Object
W3Schools: JavaScript Math Object
Pi
- Math.pi;
Round Up
- Math.ceil();
Round Down
- Math.floor();
Round to Nearest Whole Number
- Math.round();
Which Number is Smallest?
- Math.min(8, 15, 3, 63);
- → 3>
Which Number is Largest?
- Math.max(8, 15, 3, 63);
- → 63>
Random Number (0-1)
- Math.random();>
- Returns a random number between 0 (inclusive) & 1 (exclusive)-not including 1
- Always returns a number lower than 1
- 16 decimal places long
Random Number--Range from 0 to anything you want
Math.random() used with Math.floor() can be used to return random integers.
- Math.floor(Math.random() * 9)+1;>
- → Returns a random integer from 0 to 9
- Math.floor(Math.random() * 10)+1;>
- → Returns a random integer from 0 to 10
- Math.floor(Math.random() * 100)+1;>
- → Returns a random integer from 0 to 100
typeof Operator
You can use the JavaScript typeof (notice not camelCase) operator to find the type of a JavaScript variable.
The typeof operator returns the type of a variable or an expression as a string:
- typeof "John" → "string"
- typeof 314 → "number"
OR typeof < variable name>
- const num = 5;
- typeof num; → "number"
ParseInt() & ParseFloat() Functions
GeeksForGeeks: JavaScript parseInt() Function
W3Schools: JavaScript parseFloat() Function
Use to parse strings into numbers, but watch out for Nan!
parseInt()
The parseInt() function is used to accept the string, radix parameter and convert it into an integer. If the string does not contain a numeric value then it returns NaN.
Syntax:
- parseInt(Value, radix)
- Parameters: This function accepts two parameters as described below:
- Value: This parameter contains a string which is converted to an integer.
- radix: This parameter represents the radix, or base, to be used and is optional.
- Return Value: It returns a number, and if the first character can't be converted to a number then the function returns NaN. It actually returns a number parsed up to the point where it encounters a character that is not a number in the specificed radix(base).
Examples:
- parseInt('24') → 24
- parseInt('24.8392') → 24
- parseInt('28dayslater') → 28
parseFloat()
The parseFloat() function parses a string and returns a floating point number.
- This function determines if the first character in the specificed string is a number. If it is, it parses the string until it reaches the end of the number, and returns the number as a number, not as a string.
- Note: Only the first number in the string is returned!
- Note: Leading and trailing spaces are allowed
- Note: If the first character cannot be converted to a number, parseFloat() returns NaN.
Examples:
- parseFloat('24.987') → 24.987
- parseInt('7') → 7
- parseInt('I ate 3 shrimp') → NaN
If, Elseif, Else Statements
- Use if to specify a block of code to be executed if a specified condition is true.
- Use else if if the first condition is false, this is a new conditional and block of code to be executed if this one is true. You can have multiple else if's in one statement.
- Use else to specify a block of code to be executed if all of the previous conditionals were false. Don't add anything into a parameter for this one.
If/Else Example: (using template literals)
-
<script>
let highScore = 1430;
let userScore = 1600;
if (userScore >= highScore) {
console.log(`Congrats, you have the new high score of ${userScore}`);
highScore = userScore;
} else {
console.log(`Good Game. Your score of ${userScore} did not beat the high score of ${highScore} this time.`); }
</script>
Nesting Conditionals
You can nest conditional inside of conditionals.
Example:
- let password = "cat dog";
- if (password.length >= 6) {
- if (password.indexOf(' ') === -1) {
(Checking for spaces: -1 means it does not contain spaces, index position indicated if it does)
- console.log("Valid password");
- }
- else {
- console.log("Password cannot contain spaces");
- }
- }
- else {
- console.log("Password too short");
- }
Switch Statements in JavaScript
W3Schools: JavaScript Switch Statement
The switch statement is used to perform different actions based on different conditions.
You can use the switch statement to select one of many code blocks to be executed.
Syntax
- switch (expression) {
case x:
// code block when result of expression matches x
break;
case y:
// code block when result of expression matches y
break;
default:
// code block when none of the values match the value of the expression
}
Example:
- var month = 'December';
- switch(month) {
case 'July':
document.write("Happy July 4th!");
break;
case 'November':
case 'December':
document.write("Merry Christmas!");
break;
default:
document.write("The month is " + month);
}
→ Merry Christmas
You can list two cases in a row if they have the same output (Nov. & Dec.)
default statement is in case there is no match to switch statement
break is used to stop the statement from continuing to run
Ternary Operator
You can turn an if/else statement into one line of code.
Syntax:
- condition ? expIfTrue: expIfFalse
Example using if/else:
- let num = 7;
if (num === 7) {
console.log('lucky');
}
else {
console.log('unlucky');
}
Example using Ternary Operator:
- let num === 7 ? console.log('lucky'): console.log('unlucky');
JavaScript Dates
To print current date and time:
- var showDate = new Date();
document.write(showDate); - → Tue Oct 09 1018 19:27:41 GMT-0500(CDT)
Get Methods
- var date = new Date();
- var dayOfMonth = date.getDate();
- var dayOfWeek = date.getDay();
- var month = date.getMonth();
- var year = date.getFullYear();
- var hours = date.getHours();
- var minutes = date.getMinutes();
- var seconds = date.getSeconds();
- document.write("Today's date is: "< br>"
+ "Day: " + dayOfWeek + "< br>"
+ "Month: " + month + "< br>"
+ "Year: " + year); - → Today's date is:
Day: 2 → Tuesday
Month: 9 → October
Year: 2018 → 2018
Set Method
You can set a date to show whatever you'd like:
- var setDate = new Date();
setDate.setMonth(11);
setDate.setFullYear(2025); - document.write(setDate);
→ Wed Dec 09 2025 19:57:42
Adding Audio using JavaScript
W3Schools: HTML DOM Audio Object
W3Schools: Audio play() Method
Syntax
- var audio = new Audio('sounds/audio_file.mp3');
- audio.play();
Example:
- document.querySelectorAll(".drum") .addEventListener("click", function() {
var audio = new Audio('sounds/tom-1.mp3');
audio.play();
});
Additional Sound Controls
- The play() method starts playing the current audio
- There is also a pause() method.
- Use the controls property to display audio controls (like play, pause, seeking, volume, etc. attached on the audio)
JavaScript Arrays
W3Schools: JavaScript Array Reference
Arrays are ordered collections of data:
- var friends = ["Charlie", "Liz", "David", "Mattias"];
Syntax:
- Use square brackets [ ] with individual items separated by a comma.
Arrays are indexed starting at 0. Every slot has a corresponding number:
Charlie | Lizzy | David | Mattias |
0 | 1 | 2 | 3 |
We use these indices to retrieve data:
- var friends = ["Charlie", "Liz", "David", "Mattias"];
- console.log(friends[0]);
- → "Charlie"
- friends[1] + "<3 " + friends[2]
- → "Lizzy <3 David"
We can also update arrays:
- var friends = ["Charlie", "Liz", "David", "Mattias"];
- friends[0] = "Chuck";
- friends[3] = "Matt";
Chuck | Lizzy | David | Matt |
0 | 1 | 2 | 3 |
We can also add new data:
- var friends = ["Chuck", "Liz", "David", "Matt"];
- friends[4] = "Emily";
Chuck | Lizzy | David | Matt | Emily |
0 | 1 | 2 | 3 | 4 |
We can initialize an empty array two ways
- var friends = [];
- → no friends added yet
- var friends = newArray();
- → this is uncommon
Arrays can hold any type of data:
- var randomCollection = [49, true, "David"];
Nested arrays:
- const gameBoard = [
- ['X', null, 'O']
- [null, 'X', 'O']
- ['X', 'O', null]
- ];
To access add an X to the middle row to win, you can chain indeces positions together:
- animalPairs[1] → This will give you the entire second array [null, 'X', 'O']
- animalPairs[2][0] → This will give you only null
- animalPairs[2][0] = 'X'
Array Methods
Simple Steps Code: How to Remember the Difference between Unshift/Shift & Push/Pop
Unshift/Shift & Push/Pop: The one with the longer word makes the array longer
Unshift()
Use unshift() to add to the front of an array:
- var colors = ["red", "orange", "yellow"];
- colors.unshift("infared");
- → ["infared", "red", "orange", "yellow"]
Shift()
Use shift() to remove the first item of an array:
- var colors = ["red", "orange", "yellow"];
- colors.shift();
- → ["orange", "yellow"]
You can also use shift() and save the removed data in a variable:
- var colors = ["red", "orange", "yellow"];
- var col = colors.shift();
- → shift(); returns the removed element "red"
Push()
Use push() to add to the end of an array:
- var colors = ["red", "orange", "yellow"];
- colors.push("green");
- → ["red", "orange", "yellow", "green"]
Pop()
Use pop() to remove the last item of an array:
- var colors = ["red", "orange", "yellow"];
- colors.pop();
- → ["red", "orange"]
You can also use pop() and save the removed data in a variable:
- var colors = ["red", "orange", "yellow"];
- var col = colors.pop();
- → pop(); returns the removed element "yellow"
Concat()
Use concat() to merge two or more arrays. This method does not change the existing arrays, but instead returns a new array:
- const array1 = ['a', 'b', 'c'];
- const array2 = ['d', 'e', 'f'];
- console.log(array1.concat(array2));
- → Array ['a', 'b', 'c', 'd', 'e', 'f']
- It is in this order because array1 was concatenated with array2. Could be reversed if listed that way in concatenation.
Includes()
Use includes() to search for an element inside of an array:
- var fruit = ['banana', 'orange', 'lemon', 'apple', 'mango'];
- fruit.includes('lemon');
Returns a boolean: either true or false
- → true
indexOf()
Use indexOf() to find the index of an item in an array. Works just like str.indexOf():
- var friends = ["Chuck", "Liz", "David", "Matt", "Liz"];
Returns the first index at which a given element can be found:
- friends.indexOf("David");
- → 2
- friends.indexOf("Liz");
- → 1 - not 4
Returns -1 if the element is not found:
- friends.indexOf("Hagrid");
- → -1
Join()
Use join() to create and return a new string by concatenating all of the elements in an array, separated by commas or a specified separator string. If the array has only one item, then that item will be returned without the separator:
- let elements = ['Fire', 'Air', 'Water'];
Without a specificed separator (uses a comma as default):
- console.log(elements.join());
- → "Fire,Air,Water"
With a space as a separator:
- console.log(elements.join(' '));
- → "Fire Air Water"
With a dash as a separator:
- console.log(elements.join('-'));
- → "Fire-Air-Water"
With no separator (empty string):
- console.log(elements.join(''));
- → "FireAirWater"
Length
Use length() to return the number of elements in an array:
- var fruit = ['banana', 'orange', 'lemon', 'apple', 'mango'];
- console.log(fruit.length);
- → 5
Slice
Use slice() to copy parts of an array:
- var fruit = ['banana', 'orange', 'lemon', 'apple', 'mango'];
Use slice() to copy the 2nd and 3rd fruits:
- Specify index where the new array starts (1) and ends (3)
- var citrus = fruit.slice(1,3);
- citrus → ['orange', 'lemon']
- This does not alter the original fruit array
You can also use slice() to copy from a starting point and go until the end. For this you will only explicitly state the starting point:
- var birds = ['cardinal', 'bluebird', 'rooster', 'robin'];
- var favoriteBirds = birds.slice(2);
- favoriteBirds → ['rooster', 'robin']
You can also use slice() to copy from end counting backward. For this you will list a negative number of how many items you would like (but still lists them in forward-order):
- var birds = ['cardinal', 'bluebird', 'rooster', 'robin'];
- var favoriteBirds = birds.slice(-3);
- favoriteBirds → ['bluebird', 'rooster', 'robin']
You can also use slice() to copy an entire array:
- var nums = [1, 2, 3];
- var otherNums = nums.slice();
- nums → [1, 2, 3]
- otherNums → [1, 2, 3]
Splice
Use splice() to delete, replace or add new elements in an array:
Syntax:
- varName.splice(start index, how many to delete, item to add);
Beginning code:
- let animals = ['cat', 'dog', 'lizard', 'bird'];
Add in 'octopus' after 'dog'
- animals.splice(2,0,'octopus');
- animals = ['cat', 'dog', 'octopus', 'lizard', 'bird'];
Delete 'lizard' from original array
- animals.splice(2,1)
- animals = ['cat', 'dog', 'bird'];
Replace 'bird' with 'quail' from original array
- animals.splice(3, 1, 'quail');
- let animals = ['cat', 'dog', 'lizard', 'quail'];
Sort
Use sort() to sort an array. It does sort alphabetically, but not numerically (unless you pass in a function). This will change the originial array, not create a new one:
Reverse
Use reverse() to reverse the order of elements in an array. This will change the orginial array, not create a new one:
- var fruit = ['banana', 'orange', 'lemon', 'apple', 'mango'];
- fruit.reverse();
- → ['mango', 'apple', 'lemon', 'orange', 'banana'
JavaScript Objects
Objects are collections of properties. Properties are a key-value pair. Rather than accessing data using an index, we use custom keys.
Store data in key-value pairs. Written as name:value (name and value separated by a colon).
These are useful because it helps you group your code together, and it helps you avoid name-space collision (naming similar items with same name).
Object Literal: Just Like an Array
Object Literal Syntax:
- All values contained within a set of curly braces
- A colon separates property name from value
- A comma separates each name-value pair from the next
- There should be no comma after the last name-value pair
Standard JavaScript naming conventions still apply - (camelCase, don't start with numbers, etc.)
Tap into the properties within an object using dot notation (phone.model) or (phone.features.bluetooth)
- var phone = {
manufacturer: "Apple",
model: "iPhone 7",
color: "white",
features: {
bluetooth: true,
wifi: true,
gps: false
}
};
console.log(phone.features.wifi); - → true
Object: Using brackets
- var phone = new Object();
phone["manufacturer"] = "Apple";
phone["model"] = "iPhone";
phone["color"] = "silver";
console.log(phone); - → Object
manufacturer: "Apple"
model: "iPhone"
color: "silver" - OR
console.log(phone["model"]); - → "iPhone"
Object: Using Dot(.) Method
- var phone = new Object();
phone.manufacturer = "Apple";
phone.model = "iPhone 6";
phone.color = "black";
console.log(phone);
Retrieving Data in Objects
You have two choices: bracket and dot notation
- Bracket notation, similar to arrays:
- console.log(phone["model"]);
- Dot notation
- console.log(phone.model);
There are some differences between the two
- You cannot use dot notation if the property starts with a number
- someObject.1blah → Invalid
- someObject["1blah"] → Valid
- You cannot use dot notation for property names with spaces
- someObject.fav color → Invalid
- someObject["fav color"] → Valid
- You can search using a variable with bracket notation
- var str = "name";
- someObject.str → Doesn't look for "name"
- someObject[str] → Does evaluate str and looks for "name"
Update Data inside an Object
Just like an array: access the property and reassign it
- var person = {
name: "Travis",
age: 21,
city: L.A.
};
To update age:
- person["age"] += 1;
To update city:
- person.city = "London";
Updated object:
- var person = {
name: "Travis",
age: 22,
city: London
};
Object Methods (Function inside of an object)
A method is a function stored as a property
- var person = {
name: "Travis",
age: 21,
city: L.A.
friends: ["Bob", "Tina"],
add: function(x,y) {
return x + y;
}
}
The method is add: function(x, y) and line below
To call it: person.add(10,5); → 15
Object with an Added Function Below
- var phone = {
manufacturer: "Apple";
model: "iPhone 7";
color: "white";
features: {
bluetooth: true,
wifi: true,
gps: false,
}
};
function yourPhone() {
alert("Manufacturer: " + phone.manufacturer);
}
yourPhone(); - → Manufacturer: Apple
Objects: Constructor Functions
For these the names of the function have to be Capitalized on the first letter. This is how you can tell it is a constructor function, and not just any function.
- It will accept inputs (in parantheses). These are the inputs that we will provide when we create new objects.
- We match the inputs to the property names
- function BellBoy (name, age, hasWorkPermit, languages) {
this.name = name;
this.age = age;
this.hasWorkPermit = hasWorkPermit;
this.languages = languages;
this.moveSuitcase = function() {
alert("May I take your suitcase?");
pickUpSuitcase();
move();
}
Initialize New Object with Constructor Function
Don't forget: keyword new and Capitalized Function Name
This new object uses the function from above:
- var bellBoy1 = new BellBoy("Timmy", 19, true, ["French", "English"]);
Call the method inside the object
- bellBoy1.moveSuitcase();
While Loops
Repeat code while a condition is true
While loops are useful for situations where you don't know how many times the loop will run. For example: during a game it will run until the game is over.
Example: Check to see if a user is alive in game
It is very similar to an if statement, except it repeats a given code block instead of just running it once
- while(someConditionIsTrue) {
- //run some code
- //increment here
- }
Example: Print numbers from 1-5
- var count = 1;
- while (count < 6) {
- console.log("Count is: " + count);
- count++;
- }
- → Count is: 1
- → Count is: 2
- → Count is: 3
- → Count is: 4
- → Count is: 5
You can use count +=2 if you want it to count by 2's
Example: Print each character in a string
String we're looping over:
- var str = "hello";
First character is at index 0
- var count = 0;
- while(count < str.length) {
- console.log(str[count]);
- count++;
- }
- → "h"
- → "e"
- → "l"
- → "l"
- → "o"
Infinite Loops
Infinite loops occur when the terminating condition in a loop is never true
- var count = 0;
- while (count < 10) {
- console.log(count);
- }
The above example prints "0" over and over again because count is never incremented
For Loops
For Loops: another way of repeating code
Example: Used to iterate over a loop a specific number of times
Syntax:
- for (var starting count; ending count; step/what kind of change){
- //run some code
- }
Example: Print numbers from 1-5
- for (i=1; i<6; i++) {
- console.log(count);
- }
- → 1
- → 2
- → 3
- → 4
- → 5
Example: Print each character in a string
- var str = "hello";
- for (i=0; i< str.length; i++) {
- console.log(str[i]);
- }
- → h
- → e
- → l
- → l
- → o
Example: Reverse order of string
- let word = 'stressed';
- let reversedWord = '';
- for (let i = word.length-1; i>=0, i--) {
- reversedWord = reversedWord + word[i];
- }
- → desserts
Example: Using String Template Literal
Print Perfect Square Sequence
- for (let i=1; i<=10; i++) {
console.log(`${i}x${i} = ${i * i}`);
}
- → 1x1 = 1
→ 32 2x2 = 4
→ 32 3x3 = 9
→ 32 4x4 = 16
→ 32 5x5 = 25
→ 32 6x6 = 36
→ 32 7x7 = 49
→ 32 8x8 = 64
→ 32 9x9 = 81
→ 32 10x10 = 100
Nested For Loops
A nested for loop runs the outside loop once, then completes the entire inside loop before looping back to the outside one for one run through.
- for (let i = 1; i<= 3; i++) {
- console.log('OUTER LOOP:', i);
- for (let j = 3; j>=0, j--) {
- console.log(' INNER LOOP', j);
- }
- }
- → OUTER LOOP: 1
→ INNER LOOP 1
→ INNER LOOP 0
→ OUTER LOOP: 2
→ INNER LOOP 1
→ INNER LOOP 0
For...of Loops
Creates a loop iterating over iterable objects. It invokes a custom iteration hook with statements to be executed for the value of each distinct property of the object.
Does not work in Internet Explorer
Syntax:
- for (variable of iterable) {
- statement
- }
- variable: on each iteration a value of a different property is assigned to variable. variable may be declared with const, let, or var
- iterable: object whose iterable properties are iterated
Example written with regular for loop:
- let subreddits = ['soccer', 'popheads', 'cringe', 'books'];
- for (let i = 0; i < subreddits.length; i++) {
- console.log(subreddits[i]);
- }
Same example using for...of:
- let subreddits = ['soccer', 'popheads', 'cringe', 'books'];
- for (let new of subreddits) {
- console.log(new);
- }
Another example using for...of
- for (let char of 'cockadoodledoo') {
- console.log(char.toUpperCase());
- }
For...in Loops
This iterates over all enumerable properties of an object that are keyed by strings.
Syntax:
- for (variable in object) {
- statement
- }
- variable: a different property name is assigned to variable on each iteration.
- object: object whose non-Symbol enumerable properties are iterated over
Coding Challenges
Challenge: Switch two values without typing numbers or redeclaring variables.
- var a="Cat";
- var b="Dog";
- Answer:
- var c=a;
- a=b;
- b=c;
- → a="Dog", b="Cat"
Challenge: String Length Property
Create a text input that pops up when users enters your site. Ask them a question which allows them to have a 140 character answer. After they enter their answer tell them how many characters they have used, and how many they have left.
- Answer: var answer = prompt("How are you today? (Limit 140 characters)");
var currentNumber = answer.length;
var numberLeft = 140 - answer.length;
alert("You have written " + currentNumber + " characters. You have " + numberLeft + " characters left.");
Challenge: Use .Slice
Slice User Input to Specific Length
Create a text input that pops up when user enters your site. Ask them what their tweet is, which allows them to have a 140 character answer. After they enter their answer, slice it to 140 characters and show them what their tweet will look like after being sliced.
- Answer: var answer = prompt("How are you today? (Limit 140 characters)");
var tweet = answer.slice(0,140);
alert("Here is your tweet: " + tweet);
Challenge: Change text case
Ask for User's Name, return "Hello, (name)!" with capital first letter (no matter what was entered). Can only use .toUpperCase, .toLowerCase, .slice and .length.
- var name = prompt("What is your name?");
var sliceFirstLetter = name.slice(0,1);
var capitalFirstLetter = sliceFirstLetter.toUpperCase();
var restOfName = name.toLowerCase();
var sliceRestOfName = restOfName.slice(1,name.length);
var capitalizedName = capitalFirstLetter + sliceRestOfName;
alert("Hello, " + capitalizedName + "!");
Challenge: Bottles of Milk
Write function that can tell you how many bottles of milk ($1.50 each) you can buy with the amount of money passed in.
This answer also uses mod to round down to the nearest whole number.
- function getMilk (dollars) {
console.log("Buy " + ((dollars - (dollars % 1.5)) / 1.5) + " bottles of milk.");
}
getMilk(5);
This answer uses math.floor instead (which is a better way of doing it).
- function getMilk (dollars) {
console.log("Buy " + (Math.floor(dollars / 1.5)) + " bottles of milk.");
}
getMilk(8);
→ Buy 5 bottles of milk.
Challenge: Bottles of Milk, cont.
Same as above, but add to code so that it returns how much money is left over after buying the milk. Save the return in a var called moneyLeftover.
- function getMilk (dollars) {
console.log("Buy " + (Math.floor(dollars / 1.5)) + " bottles of milk.");
return dollars % 1.5; //this line says dollars mod 1.5(price of a bottle) to get the leftover amount
}
var moneyLeftover = getMilk(4);
→ Buy 2 bottles of milk.
moneyLeftover
→ 1
Challenge: BMI Calculator
Write a function that has two inputs (weight & height, in that order) and one output (calculated BMI). The output should be rounded to the nearest whole number. Don't write any alerts, prompts or console.logs. Code should only contain the function, and the result has to be returned by the function. You do not need to call the function. Hint: Math.round(). Formula: BMI = weight(kg) / height2(meters2).
- function bmiCalculator (weight, height) {
return Math.round(weight / (Math.pow(height,2)));
}
var bmi = bmiCalculator(65,1.8);
→ 20
Challenge: Love Calculator
Write two prompts that ask for two names of people. Then have a random number generator create a random number from 0-100, and alert their love score in percentage.
- var name1 = prompt ("What is your name?");
var name2 = prompt ("Who do you want your love score with?");
var randomScore = Math.floor(Math.random() *101);
alert (name1 + " and " + name2 + "'s love score is " + randomScore + "%!");
Challenge: Love Calculator, cont.
Same as above, adding conditionals.
- var name1 = prompt ("What is your name?");
var name2 = prompt ("Who do you want your love score with?");
var randomScore = Math.floor(Math.random() *101);
if (randomScore > 75) {
alert (name1 + " and " + name2 + "'s love score is " + randomScore + "%! Sounds like a match made in heaven!");
} else {
alert (name1 + " and " + name2 + "'s love score is " + randomScore + "%!");
}
Challenge: Love Calculator, cont.
Same as above, adding conditionals with comparison & logical operators
- var name1 = prompt ("What is your name?");
var name2 = prompt ("Who do you want your love score with?");
var randomScore = Math.floor(Math.random() *101);
if (randomScore > 70) {
alert (name1 + " and " + name2 + "'s love score is " + randomScore + "%! Sounds like a match made in heaven!");
}
if (randomScore >30 && randomScore <=70){
alert (name1 + " and " + name2 + "'s love score is " + randomScore + "%! That's a pretty good score!");
}
if (randomScore <= 30) {
alert (name1 + " and " + name2 + "'s love score is " + randomScore + "%! Hmm...maybe it's not meant to be.");
}
Challenge: BMI Calculator, cont.
Advanced-Calculate user's BMI as before, but add messages about what their result means. Write a function that outputs(returns) a different message depending on the BMI. The message must be returned as an output from your function. You should not need to use alert, prompt or console.log in this challenge.
- function bmiCalculator (weight, height) {
var bmi = Math.round(weight / (Math.pow(height,2)));
if (bmi > 24.9) {
return ("Your BMI is " + bmi + ", so you are overweight.");
}
if (bmi >= 18.5 && bmi <= 24.9) {
return ("Your BMI is " + bmi + ", so you have a normal weight.");
}
if (bmi < 18.5) {
return ("Your BMI is " + bmi + ", so you are underweight.");
}
}
Challenge: Leap Year (Difficult!)
Write a program that works out whether if a given year is a leap year. A normal year has 36t5 days, lear pyears have 366, with an extra day in February. This is how to work out whether if a particular year is a leap year:
A year is a leap year if it is evenly divisible by 4;
except if that year is also divisible by 100;
unless that year is also evenly divisible by 400.
Hints: Remember that the modulo operator gives you the remainder of a division. Try to visualize the rules by creating a flow chart.
- function isLeap (year) {
if (year %4 !== 0)
return ("Not leap year.")
if (year %4 === 0 && year %100 !== 0)
return ("Leap year.")
if (year %4 === 0 && year %100 === 0 && year %400 !==0)
return ("Not a leap year.")
if (year %4 === 0 && year %100 === 0 && year %400 ===0)
return ("Leap year.")
}
Challenge: Guest List (Array: Includes)
Write a guest list for a party. When a person comes to the door for the party ask them to type in their name. Have the program check the guest list for their name, and if it is there alert, "Welcome", and if it doesn't alert ,"Sorry, maybe next time".
- var guestList = ["Angela", "Jack", "Pam", "James", "Laura", "Jason"];
var name = prompt("What is your name?");
if (guestList.includes(name) === true) {
alert ("Welcome!");
} else {
alert ("Sorry, maybe next time!")
}
Challenge: FizzBuzz
Write a program that prints the numbers from 1 to 100. But for multiples of three print, "Fizz" instead of the number, and for multiples of five print, "Buzz". For numbers which are multiples of both three and five print, "FizzBuzz".
- var output = [];
var count = 1;
function fizzBuzz() {
if ((count %3 ===0) && (count %5 ===0)) {
output.push("FizzBuzz");
} else if (count %3 ===0) {
output.push("Fizz");
} else if (count %5 ===0){
output.push("Buzz");
} else
output.push(count);
count++;
console.log(output);
}
Challenge: FizzBuzz, cont. - Using While Loop
Take the code from FizzBuzz and add a while loop to make it run automatically until count=100.
- var output = [];
var count = 1;
function fizzBuzz() {
while (count <= 100) {
if ((count %3 ===0) && (count %5 ===0)) {
output.push("FizzBuzz");
} else if (count %3 ===0) {
output.push("Fizz");
} else if (count %5 ===0){
output.push("Buzz");
} else
output.push(count);
count++;
}
console.log(output);
}
Challenge: FizzBuzz, cont. - Using For Loop
Take the code from the FizzBuzz while loop and refactor it into a for loop.
- var output = [];
function fizzBuzz() {
for (var count = 1; count <= 100); count++) {
if ((count %3 ===0) && (count %5 ===0)) {
output.push("FizzBuzz");
} else if (count %3 ===0) {
output.push("Fizz");
} else if (count %5 ===0){
output.push("Buzz");
} else
output.push(count);
}
console.log(output);
}
Challenge: Who's Buying Lunch?
You are going to write a function which will select a random name from a list of names. The person selected will have to pay the bill for everybody's food. Important: the output should be returned from the function and you do not need alert, prompt or console.log. Hints: You might need to think about array.length. Remember that arrays start at position 0.
- function whosPaying(names) {
names = ["Angela", "Ben", "Jenny", "Michael", "Chloe"];
var random = Math.floor(Math.random() * names.length);
return (names[random] + " is going to buy lunch today!");
}
This is an alternative way to write the code that is also correct:
- function whosPaying(names) {
names = ["Angela", "Ben", "Jenny", "Michael", "Chloe"];
var random = names[Math.floor(Math.random() * names.length)];
return (random + " is going to buy lunch today!");
}
This is yet another alternative way to write the code that is also correct:
- function whosPaying(names) {
names = ["Angela", "Ben", "Jenny", "Michael", "Chloe"];
var numberOfPeople = names.length
var randomPersonPosition = Math.floor(Math.random() * numberOfPeople);
var randomPerson = names[randomPersonPosition];
return randomPerson + " is going to buy lunch today!";
}
Challenge: Secret Number
Write a program that has a secret number. Prompt the user to enter a number 1-10 to guess the secret number. If they are correct let them know. Also help them by adding messages if their guess is too low or too high.
- var secretNumber = 6;
var userGuess = prompt("Guess a number 1-10");
var guess = Number(userGuess);
if (guess === secretNumber) {
alert("You're spot on!");
} else if (guess < secretNumber) {
alert("Too low. Try again!");
} else {
alert("Too high. Try again!");
}
By adding in the line with the Number() it takes a string and converts it into a number.
Challenge: 99 Bottles of Beer
Create a function that works when you call it so that it prints out all of the lyrics to the entire song.
-
function beer(count){
while (count >=0) {
if (count >= 1) {
console.log (count + " bottles of beer on the wall, " + count + " bottles of beer. Take 1 down, pass it around, " + (count - 1) + " bottles of beer on the wall.");
} else {
console.log ("No bottles of beer on the wall, no bottles of beer. Go to the store, buy 99 more, 99 bottles of beer on the wall.");
}
count--;
}
console.log(beer);
}
Challenge: The Fibonacci Exercise
Fibonacci was an Italian mathematician who came up with the Fibonacci sequence:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144... where every number is the sum of the two previous ones.
Create a function where you can call it by writing the code: fibonacciGenerator (n) where n is the number of items in the sequence. So I should be able to call fibonacciGenerator (3) and get [0,1,1] as the output. Hint: a flowchart of the logic can be extremely useful.
-
function fibonacciGenerator (n) {
var output = [0,1];
if (n == 1) {
return[0];
} else if (n == 2) {
return[0,1];
} while (output.length < n) {
output.push(output[output.length - 1] + output[output.length -2]);
}
return output;
}
Challenge: Simple Function to Check for Validity of Password
Write a isValidPassword function. It accepts 2 arguments: password and username.
Password must:
- - be at least 8 characters
- - cannot contain spaces
- - cannot contain the username
If all requirements are met, return true. Otherwise: false.
Test code:
- isValidPassword('89Fjj1nms', 'dogLuvr'); //true
- isValidPassword('dogLuvr123!', 'dogLuvr') //false
- isValidPassword('hello1', 'dogLuvr') //false
- function isValidPassword(password, username) {
- if ((password.length < 8) || (password.indexOf(' ') > -1) || (password.indexOf(username) > -1)) {
- console.log(`Sorry ${username}. Check requirements and try again.`);
- }
- else {
- console.log(`Welcome ${username}`);
- }
- }
The indexOf requirements in this function say that it is greater than -1. This is because if it returns -1 it does not exist. Specifically this is saying if a space is found or the username is found within the password then it is an invalid password.